Introduction
Forms are divided in several packages, each covering a specific part of the logic:
@banquette/form
(discussed here): contains the base logic and objects. That's where the hierarchy of components is created and where the state management is done. That's the "glue" binding other packages together.@banquette/model-form
(doc): that's the bridge between basic forms and forms defined through a model class and decorators.@baquette/vue-ui
(doc): that's where the visual part of the form is located. Forms are thought to be framework-agnostic, so the view part is separated from the base logic.Banquette
comes with a built-in Vue 3 integration.
Here we'll discuss the @banquette/form
part only.
What's a form?
A form in Banquette
is composed of two types of objects:
- controls: a control is a form field, it has a visual representation on the page and contains a value. It can be a text input, a select list, a date picker, anything you want.
- groups: a group is a collection of groups or controls. There are two types of groups: objects and arrays.
If we put it into a schema, it gives us:
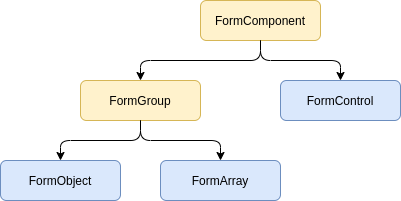
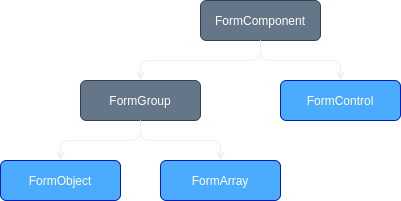
Only objects in blue are concrete classes you can use.
So, in a nutshell, a form is a tree of FormControl
, FormObject
or FormArray
.
Each of these components have a set of states, a value, a validator and potentially validation errors.
Common properties
Before digging in how it works, here is a quick overview of the properties that are common to every type of form component. They are divided in several categories to be easier to read.
Base properties
Property | Description |
---|---|
id | Unique numerical id of the component. |
formId | Extended unique id, guaranteed to be unique between forms and usable as id in the DOM. |
name | The name of the component.null for the root node, because the name of a component is given by its parent.The name of the root node is the name of the variable holding it. |
path | Absolute path to the root of the form. The path of the root component is / . If the root has a child username , its path will be /username . |
value | Form controls have the value given by their view. From groups have the combined values of their children. |
parent | Parent form component or null if none. |
root | The root component of the form. |
activeControl | The form control currently on focus. |
size | Get the "size" of the component. Meaning the number of direct children + 1 to account for the component on which the call is made. |
sizeDeep | Same as size but add to length of children instead of them counting for one. |
activeStates | The list of the active states for the component. |
disabled | A disabled component is non-interactive and excluded from the validation. |
enabled | Inverse of disabled . |
Validation related
The validation has a dedicated section that you can check out to get more information about how it works.
Property | Description |
---|---|
valid | true if the component has no validation error. |
invalid | Inverse of valid . |
validated | A component is validated when the validation has run, no matter if errors have been found or not. |
notValidated | Inverse of validated . |
validating | A component is validating when its validator or the one of one of its children is currently running. |
notValidating | Inverse of validating . |
validatedAndValid | Only true when the component has been validated, has no validation running and no error have been found. |
validator | The validator to use to validate the current value of the component. |
validationStrategy | Define when the validation should automatically run. |
errors | The list of errors found for this component. |
errorsDeep | Flattened array of errors found on the component itself and all its children (recursively). |
errorsDeepMap | A key/value pair containing the path of each children component as index and the array of their errors as value. |
View related
Property | Description |
---|---|
busy | A component is busy when its view model or the one of its children is processing something. |
notBusy | Inverse of busy . |
dirty | A component is dirty if the user has changed its value in the UI, no matter the current value. |
pristine | Inverse of dirty . |
touched | A component is marked touched once the user has triggered a blur event on it or one one of its children. |
untouched | Inverse of touched . |
changed | A component is changed when its value is different from the initial value. |
unchanged | Inverse of changed . |
focused | A component is focused when it (or a children) is the current field on edition. |
unfocused | Inverse of focused . |
Other
Property | Description |
---|---|
concrete | A component is concrete if: - For a FormControl : it has a view model,- For a FromObject : at least one of its children is concrete,- For a FormArray : it has been accessed from the viewA virtual component is not part of the validation process nor of the exported values, so it will basically be invisible from outside of the form. Please refer to this part of the documentation if you want more information. |
virtual | Inverse of concrete . |