Form basic concepts
Before you look at how form components work, there are some basic concepts on how forms in general work in Banquette
.
Architecture
All built-in form components of Banquette
are made to work with the underlying form architecture, that we can summarize with the following diagram:
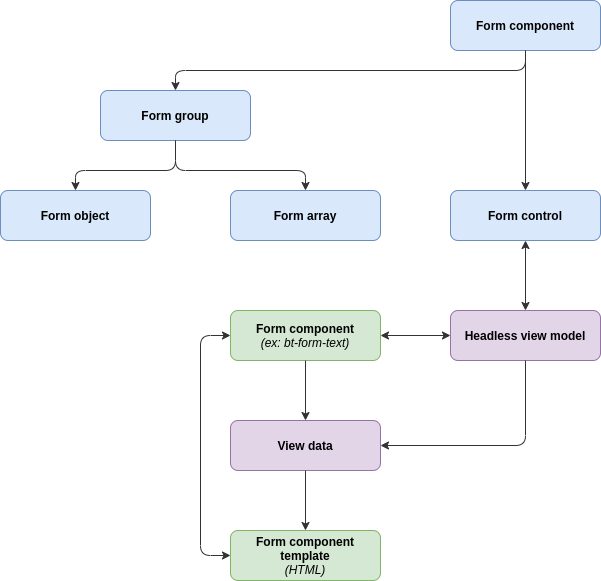
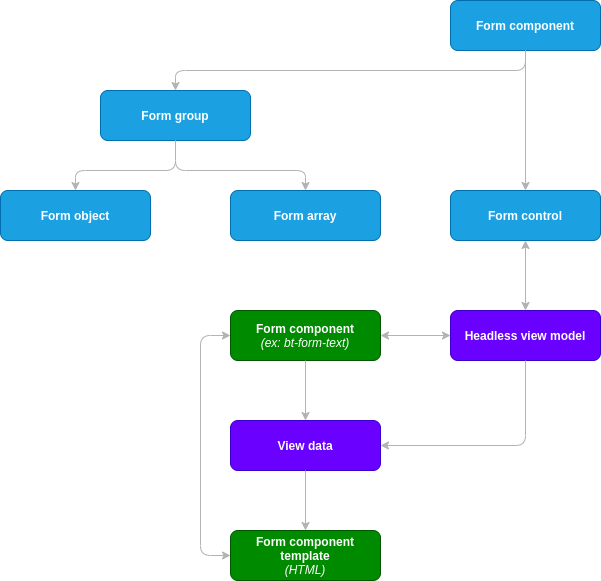
There are three part in this schema:
- In
the elements part of the Form component. These components are totally framework-agnostic and have no direct relation with the view or VueJS in general.
- In
the elements part of the Headless UI, that are meant to be framework-agnostic too.
- In
the elements part of the
VueJS
component.
To recap the role of each part:
elements responsible for managing the form's tree, states and validation.
hold most of the UI logic "framework-agnosticly". They create a
viewData
object.your typical
VueJS
component (a view model and a template).
NOTE
If you need more information on how form and the headless ui components work please refer to their dedicated documentation:
Form control
So as you can see in the diagram, any form component is associated with a FormControl
through its headless view model.
The states of the FormControl
(disabled, dirty, invalid, etc.) are synchronized with the view data object created by the view model, and that is exposed to the view.
So any form component you create must be linked to a FormControl
:
import { FormControl } from "@banquette/form";
@Component()
export default class MyComponent {
@Expose() public myControl = new FormControl();
}
<bt-form-text :control="myControl"></bt-form-text>
If a component is not associated with a FormControl
, it will remain disabled until it does.
It can happen at any time, and change over time.
You can also refer to the form control via its name in the form:
import { FormControl, FormObject } from "@banquette/form";
@Component()
export default class MyComponent {
@Expose() public form = new FormObject({
title: new FormControl(),
category: new FormObject({
name: new FormControl()
})
});
}
<bt-form-text control="title" :form="form"></bt-form-text>
<bt-form-text control="category/name" :form="form"></bt-form-text>
TIP
You can greatly simplify this notation using the bt-form component that we'll see later.
v-model
If you're familiar with the conventional way of binding a form control with a value, you might find this notion of FormControl
over-complicated.
That's why the v-model
notation is also supported. So you can also do:
The form component will handle the creation of a FormControl
for you, in the background. This means you can still attach validators to it:
NOTE
This notation is only meant for basic forms.
If you have a complex form that you need to synchronize with your server or that has dynamic parts and interactions, you should consider using bt-form instead.